Go Delphi
While working on a large distributed orchestration system, I realised that the basic cryptographic operations I needed could and should be broken out it’s own package. Go Delphi was born of the need to provide a sensible API for common cryptographic operations. Under the hood, it uses the ed25519, X25519, and ChaCha20-Poly1305 to provide fast and efficient operations, and forward secrecy to boot.
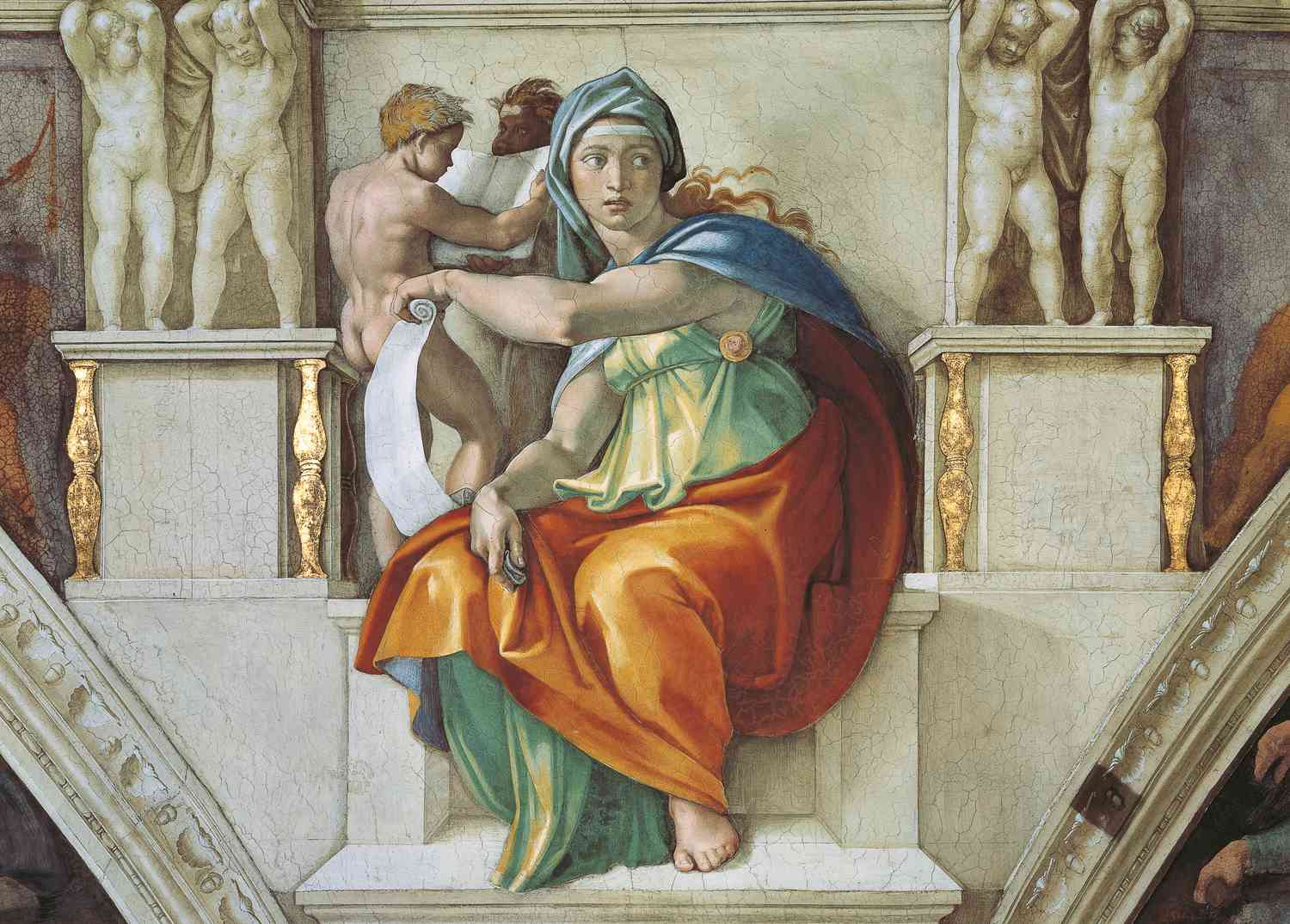
Concepts
Principals are a concept representing a holder of keys, along with the things it can do. In practice, this is an array of Keys, with some methods attached.
Keys have an encryption component and a signing component. In this system, private keys and public keys are each 64 bits.
Messages are simply structures that hold plain text, cipher text, and all inforation necessary to sign, encrypt to a recipient, verify, and decrypt.
Messages are efficiently serialized for transport using msgpack.
Getting Started
import (
"crypto/rand"
"testing"
"github.com/sean9999/go-delphi"
"github.com/stretchr/testify/assert"
)
func TestExample(t *testing.T) {
// some plain text
sentence := []byte("hello world")
// create two principals
alice := delphi.NewPrincipal(rand.Reader)
bob := delphi.NewPrincipal(rand.Reader)
// create a message for bob, from alice
msg := delphi.NewMessage(rand.Reader, sentence)
msg.Sender = alice.PublicKey()
msg.Recipient = bob.PublicKey()
// add some metadata (this becomes AAD)
msg.Headers.Set("foo", []byte("bar"))
msg.Headers.Set("bing", []byte("bat"))
// encrypt message
err := msg.Encrypt(rand.Reader, alice, nil)
assert.NoError(t, err)
// decrpyt message
err = bob.Decrypt(msg, nil)
assert.NoError(t, err)
// is decrypted text same as plain text?
assert.Equal(t, sentence, msg.PlainText)
// has the metadata survived?
foo, ok := msg.Headers.Get("foo")
assert.True(t, ok)
assert.Equal(t, []byte("bar"), foo)
}